Course Content
1.
Course Intro
0 min
10 min
0
2.
Why Learn Python?
4 min
5 min
0
3.
What is the Best Software for Learning Python?
5 min
5 min
0
4.
Intro to Google Colaboratory
28 min
20 min
0
5.
Intro to Temzee Lessons
4 min
4 min
2
6.
How to Declare a Variable in Python
2 min
3 min
0
7.
Declare Variables Exercises
0 min
4 min
9
8.
Use Descriptive Variable Names
4 min
3 min
0
9.
Variable Naming Rules and Conventions
8 min
6 min
0
10.
The Print Function
4 min
3 min
0
11.
Integers and Floats
0 min
4 min
0
12.
Integers and Floats Exercises
0 min
1 min
4
13.
Familiar Arithmetic (math) Operators
0 min
2 min
0
14.
Math Operators Exercises
0 min
1 min
0
15.
Strings
0 min
11 min
0
16.
Comments in Python
0 min
4 min
0
17.
Functions in Python
0 min
26 min
0
18.
String Formatting with F-Strings
0 min
3 min
0
19.
Conditionals, Booleans, and If Statements
0 min
12 min
0
20.
Intro to Python Lists
0 min
6 min
0
21.
Intro to Python Lists - Exercises
0 min
2 min
6
22.
Lists as a Sequence of Values
0 min
6 min
0
23.
Coming Soon...
0 min
1 min
0

- Save
- Download
- Clear Output
- Runtime
- Run All Cells
- Difficulty Rating
Loading Runtime
Variable Naming Rules:
So far we've talked about how to store values within variables and we've talked about the importance of using descriptive variable names, but we haven't yet talked about the rules that govern the naming of variables.
Pretty much, you can't name a variable absolutely anything you want.
In Python, variable names can only be composed of the following characters:
- Upper case letters:
A-Z
- Lower case letters:
a-z
- Numbers:
0-9
- Underscores:
_
Take a second to find the underscore symbol on your keyboard. You can type an underscore by holding shift
and then pressing the -
key: _
On top of that there's one extra restriction you cannot start a variable name with a number (with a digit 0-9). You can only start a variable name with letters and underscores.
Which of the following are legal variable names in Python? Take a look through them and we'll go over them one by one:
my-name
Ryan
90_days
_internal
_
*pointer
First, my-name
. It looks natural, however, dashes -
are not allowed in variable names. If Python looks at this it's actually going to think that the dash -
is a subtraction symbol and it's going to try and subtract name
from my
and it's not going to be able to do it (unless you happen to have two other variables called my
and name
and they happen to have numeric values stored to them –in that case it would just do the subtraction). You cannot use dashes -
in variable names.
Next Ryan
with an uppercase R
. That's a good variable name. You can use both uppercase and lowercase letters.
How about 90_days
? This one doesn't work. There aren't any illegal characters in this one, but you cannot start a variable name with a number –in this case 9
.
The next two, we'll kind of talk about them together. _internal
? It looks good! It only contains letters and underscores and it doesn't start with a number. It might be a little bit odd to you that we could start a variable name with an underscore _
but it is a legal variable name.
Also, in that same vein, an underscore by itself _
is also a legal variable name. It's not a very descriptive variable name, but technically it works and there might be some rare circumstances where you would want to do that. We may talk about that in later lessons.
And last one: *pointer
? -not allowed (even though this would be allowed in some other programming languages). Asterisks are not allowed in variable names. Only underscores, letters and numbers.
Variable Names Are Case Sensitive
Ok, now that has maybe sunk in a little bit, let's try this again. I'm going to make this a little bit tricker. Maybe not harder, but trickier.
Which of the following are legal variable names in Python?
temzee
Temzee
TemZee
_temzee
__Temzee
_TEMZEE_
TEMZEE
temzee_
TEMzee
tem_zee
OK, this is kind of a trick question. Sorry to do that to you, but technically all of these are acceptable variable names in Python. Maybe not good variable names, but they are legal variable names. What I want to emphasize here is that Python will see all of these as different variables.
Variables are case sensitive in Python. So temzee
and Temzee
are two different variables. I'm sure you'll run into this issue once in a blue moon when you accidentally make something uppercase which shouldn't be, or lowercase when it shouldn't be and it will say that the variable is "undefined" or something like that.
So I wanted to make sure you knoww that variable names are case sensitive in Python.
Reserved Keywords are also not allowed
Now, before we wrap up this topic there's one more variable naming restriction that I want to tell you about. Python is known for being a really readable programming language. In fact, that's one of its great strengths. Part of what makes Python so readable is that in certain situations where other programming languages might use a funky symbol to do something, Python tends to use regular words. I mean, what a concept, right? You should be happy about this.
The thing is that this poses a slight complication for what we name our variables. The following are called "Reserved Keywords" in Python. This means that the programming language has already laid claim to all of these words and has assigned to them specific functionality within the programming language. Because of this, you can't use any reserved keywords as variable names. Python has a monopoly on them.
Reserved Keywords in Python
False | None | True | and |
as | assert | break | class |
continue | def | del | elif |
else | except | finally | for |
from | global | if | import |
in | is | lambda | nonlocal |
not | or | pass | raise |
return | try | while | with |
yield |
Now technically, you could change the casing of some of these in order to create a variable that doesn't conflict, however, I do not recommend that. As you get experience you'll start to recognize these keywords at a glance, and it will be confusing to other people if you use these words –kind of at all– as variable names. So I recommend that you just stay away from them entirely.
Another thing that I want to mention is that you absolutely do not need to memorize this list –at all. In fact, there's a somewhat easy way to tell if you're accidentally using a reserved keyword in Python.
In most code writing applications, when you type one of these reserved keywords they will show up as dark blue or purple when you write them. The application application you're using to write your code in probably has something called "syntax highlighting." –syntax highlighting is how the application color-codes the different parts of the programming language as you're writing the code. The more code you write the more familiar you'll get with this syntax highlighting.
Particularly in Google Colab and in Temzee Lessons, if you write a regular variable it will be black (in the case of Google Colab) or it will be light blue in the case of Temzee notebooks. However, in both of them, and in many other Python writing applications, if you accidentally use a reserved keyword, that reserved keyword will show up as dark blue or purple.
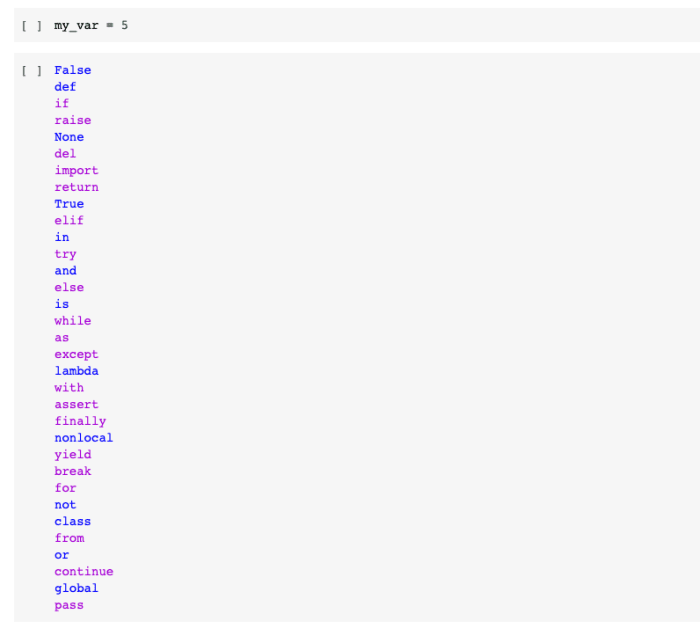
So the moral of the story is, if you're writing code and it goes funky colors, there's probably something off and you should try and double check that, but specifically with reserved keywords, remember that if it goes dark blue or purple you should double check and maybe try a different variable name.
Use snake_case
in Python
The last thing I want to share with you is not a rule about naming variables but is... [Captain Barbosa voice] –more what you'd call "guidelines" than actual rules.
I want to encourage you to use something called "snake case" when naming your variables.
Using "snake case" that means that all of the letters in our variable names are lower-case and that if a variable is made up of multiple words that we separate those words with underscores.
Now It's called snake case because if you squint hard, it kind of looks like the variable name is wiggling back and forth –up and down– kind of like you might see a snake do when it's moving.
And in fact there are other naming conventions out there that are used by other programming languages too, for example something called "camel case" is common in JavaScript programming.
camelCaseVariable
Doesn't that look just like a camel. I guess the capital letters do kind of resemble the humps on a camel's back.
Anyway, in Python, you should use snake_case
when you name your variables. And if you do, you'll fit right in with the rest of the Python community. Now there are a few instances in Python programming where using snake_case is not the convention, but do not worry about that for now. When in doubt, use snake case when naming variables in Python and you'll be good 97.2% percent of the time.